emacs-lisp-aez-notes
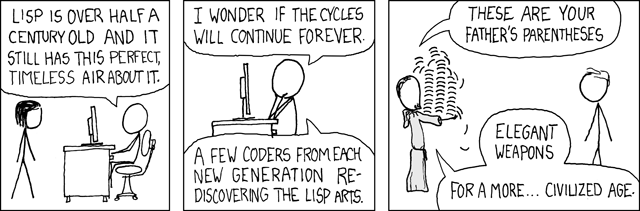
Table of Contents
Emacs Lisp the language
Variables
Use defvar
to define a variable along with optionally an initial value and a
docstring. Use setq
to set the value of an existing variable.
Nonstandard evaluation
If you want to evaulate an element of a quoted list you can use ,
as in the
following example:
`(a list of ,(+ 2 3) elements) => (a list of 5 elements)
If you want to expand a list out in a quoted list you can use ,@
as in the
following example:
(setq some-list '(2 3)) => (2 3) (cons 1 (append some-list '(4) some-list)) => (1 2 3 4 2 3) `(1 ,@some-list 4 ,@some-list) => (1 2 3 4 2 3)
Program flow
progn
to sequence commands and return the value of the last one.
String handling
The s.el
library provides sensible string functions, see the notes below.
string interpolation
(s-format "foo ${bar}" 'aget `(("bar" . ,(+ 1 1))))
Working with dates and times
format-time-string
to get datetime as a stringcurrent-time
for the current time as a list of integerstime-subtract
andtime-add
for datetime arithmetic
Working with files
Function | Use |
---|---|
directory-files |
list files in a directory |
Working with passwords
(read-passwd "magic word: ")
More details are available in the docs.
System command
To run a system command/shell command there is shell-command
.
SICP
Exercise 1.3
Define a procedure that takes three numbers as arguments and returns the sum of the squares of the two larger numbers.
Emacs Lisp Packages
JSON
For parsing and encoding to JSON there is the json.el
package.
Examples to and from a string
Open up a REPL in emacs and try the following
(json-encode '(("a" . 1) ("b" . "c"))) (json-read-from-string "{\"a\":1,\"b\":\"c\"}")
Examples reading from file
json-read-file
to read from the given file.
String
The s.el
library provides sensible string functions, see these notes for a
table of commonly used functions.
Function | Use | Example |
---|---|---|
s-format |
string interpolation | |
s-concat |
string concatenation | (s-concat "foo" "bar") |
s-join |
string concatenation (with separator) | (s-join "-" '("foo" "bar")) |
s-matches? |
regex matching |